SLTwitCtrl ActiveX Control for Twitter - Example Code
Example 1 - Visual C++ - How to
add the Twitter ActiveX control to a project
Before you can use the Twitter
component you must add the component to your Visual C++ project.
Detailed information about how to add the SLTwitCtrl ActiveX Control to
your Visual C++ project is available in this
blog post on our blog.
Example 2 - Visual C++ -
Preparing the Twitter control for communication
Before
you can send a tweet from the control to Twitter you must specify some information that
Twitter requires for communication. You must specify an application
name, a consumer key, a consumer secret, a username and a password. You
can do this by setting corresponding properties in the ActiveX control.
You can do it via the Properties window in
Microsoft Visual
C++ (as shown in the
picture to the right) or by specifying the information programmatically,
as shown in the Visual C++ example below:
|
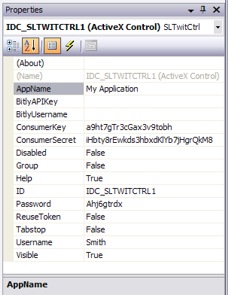 |
// Specify application name
SLTwitCtrl1.SetAppName("My Application");
//
Specify consumer key and consumer secret
SLTwitCtrl1.SetConsumerKey("a9ht7gTr3cGax3v9tobh");
SLTwitCtrl1.SetConsumerSecret(
"iHbty8rEwkds3hbxdKlYb7jHgrQkM8");
// Specify username and password
SLTwitCtrl1.SetUsername("Smith");
SLTwitCtrl1.SetPassword("Ahj6gtrdx");
|
|
A consumer key and consumer secret can be retrieved from Twitter's
website for developers. On the same website you can register your
application (which is necessary before you can send tweets to Twitter).
Open this web page
to read more.
Example 3 - Visual C++ - Send a message (a
tweet) to Twitter
This example shows how to send a
message (a tweet) to Twitter using Visual C++ code. It also shows how to handle errors, if
something goes wrong.
// Post a tweet
BOOL bStatus = SLTwitCtrl1.TwitPostMessage("This
is a tweet!");
// Check if an error occurred
if (bStatus ==
false) {
// Store error message
in this string variable
char
sErrMsg[1024];
// Get error code
long
nErrorCode = SLTwitCtrl1.TwitGetLastError();
// Create a string with
the error code
wsprintf(sErrMsg,"Error
#%d occurred!",nErrorCode);
// If error code is >
1000, Twitter may have a message for us
if
(nErrorCode > 1000) {
// Read message from Twitter
BSTR
sReplyMsg;
SLTwitCtrl1.TwitGetLastResponse(&sReplyMsg);
CString csReplyMsg =
(CString)sReplyMsg;
strcat(sErrMsg,"
Twitter returned: ");
strcat(sErrMsg,csReplyMsg.GetBuffer(1));
}
else {
// Show message box with error message
MessageBox(sErrMsg, "Error");
}
}
else {
// Show information
message box - the message want sent to Twitter
MessageBox("The
message was successfully sent to Twitter!",
"Information");
} |
Example 4 - Visual C++ - How to use
Bitly to
shorten a URL in a message
This example in Visual C++ shows
how to shorten a URL in a message by using the
TwitShortenURLInMessage method in the SLTwitCtrl ActiveX
control. The Bitly service is used.
char sMessageOriginal[1024];
' Create a message that contains a URL
strcpy(sMessageOriginal,
"Read more about MultiMailer here:
http://www.samlogic.net/multimailer/multimailer.htm");
' Now shorten the URL in the message
CString csMessageShortenedURL =
SLTwitter.TwitShortenURLInMessage("",1);
' Now display the updated message in a message box
MessageBox(csMessageShortenedURL.GetBuffer(1), "Message with
shortened URL"); |
The message text "Read more about MultiMailer here:
http://www.samlogic.net/multimailer/multimailer.htm" will be updated
to have the following contents: "You can read more about the Twitter
component here: http://bit.ly/13i0sOE". The image below shows how
the message box that is opened in the last line of the example code
above look
like:
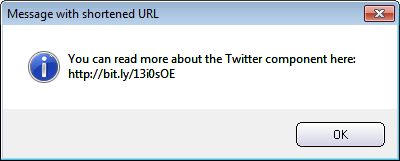
< Go back
|
|